Spring Boot Microservices
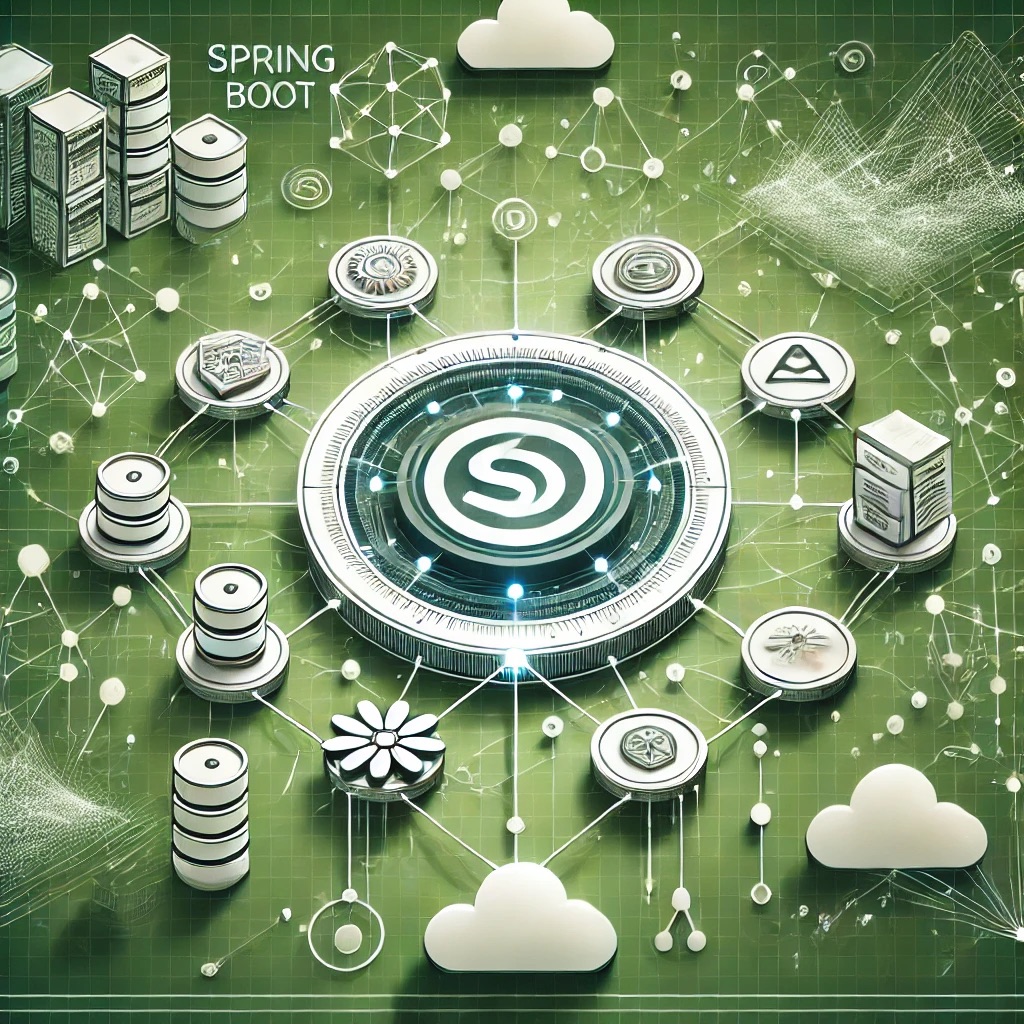
- Details
- Category: Spring Boot Microservices
- Mindful Chase By
- Hits: 110
Microservices architecture has transformed how modern software applications are designed, developed, and maintained. This article explores what microservices are, why they are essential, and how you can implement them effectively. Microservices represent a significant shift from monolithic architectures, offering scalability, maintainability, and agility.
Read more: Introduction to Microservices: What, Why, and How
- Details
- Category: Spring Boot Microservices
- Mindful Chase By
- Hits: 110
Spring Boot is a popular framework for building Java applications with minimal configuration and maximum productivity. Designed to streamline the setup and development process, Spring Boot eliminates much of the boilerplate code and complexity typically associated with traditional Spring applications. In this article, we will guide you through setting up your first Spring Boot application step-by-step.
Read more: Getting Started with Spring Boot: Setting Up Your First Application
- Details
- Category: Spring Boot Microservices
- Mindful Chase By
- Hits: 99
Building microservices with Spring Boot is an excellent way to start your journey into distributed systems. In this article, we will walk you through creating your first microservice step-by-step. You will learn how to define a simple service, connect it to a database, and expose RESTful APIs for interaction.
Read more: Building Your First Microservice with Spring Boot
- Details
- Category: Spring Boot Microservices
- Mindful Chase By
- Hits: 94
REST (Representational State Transfer) APIs are the backbone of modern web and microservice architectures. They provide a standardized way for services to communicate, making them an essential tool for building scalable and interoperable systems. This article explores the fundamentals of REST APIs, their principles, and how they are implemented in microservices.
Read more: Understanding REST APIs: The Foundation of Microservices
- Details
- Category: Spring Boot Microservices
- Mindful Chase By
- Hits: 102
Configuration management is a crucial aspect of building robust applications, especially when dealing with multiple environments such as development, testing, and production. Spring Boot simplifies configuration through the use of profiles, properties files, and YAML files, enabling developers to manage environment-specific settings with ease. In this article, we will explore these features in detail and demonstrate how to use them effectively.
Read more: Spring Boot Configuration: Profiles, Properties, and YAML Made Easy
- Details
- Category: Spring Boot Microservices
- Mindful Chase By
- Hits: 138
Dependency Injection (DI) and Inversion of Control (IoC) are foundational concepts in modern application development, especially in frameworks like Spring Boot. These principles make code more modular, testable, and maintainable by decoupling object creation from object usage. In this article, we will introduce DI and IoC, explain their significance, and demonstrate their implementation in Spring Boot.
Read more: Dependency Injection and IoC in Spring Boot: A Beginner’s Guide
- Details
- Category: Spring Boot Microservices
- Mindful Chase By
- Hits: 100
Monitoring and managing applications in production is crucial for maintaining performance, reliability, and availability. Spring Boot Actuator is a powerful tool that provides production-ready features to monitor and manage Spring Boot applications. With Actuator, you can gain insights into application health, performance metrics, and runtime behavior, all with minimal configuration.
Read more: Introduction to Spring Boot Actuator for Monitoring and Metrics
- Details
- Category: Spring Boot Microservices
- Mindful Chase By
- Hits: 103
Integrating a database with a Spring Boot application is a common requirement for modern enterprise systems. Spring Boot provides seamless integration with JPA (Java Persistence API) and Hibernate, making it easier to manage database interactions using object-relational mapping (ORM). This article explores the key concepts and steps involved in integrating a database with Spring Boot using JPA and Hibernate. Although the focus is on Spring Boot, the principles and techniques discussed can be applied across different platforms, with relevant code examples provided in C# to illustrate comparable implementations.
Read more: Database Integration with Spring Boot: Using JPA and Hibernate
- Details
- Category: Spring Boot Microservices
- Mindful Chase By
- Hits: 95
Securing microservices is a critical step in building robust and reliable systems. With the rise of distributed systems and APIs, ensuring secure communication between services and protecting sensitive data is more important than ever. Spring Security and OAuth2 are powerful tools that simplify the process of securing microservices, providing a wide range of features for authentication and authorization. This article explores how to secure microservices using Spring Security and OAuth2, offering practical insights and equivalent examples in C# to illustrate cross-platform approaches.
Read more: Securing Microservices with Spring Security and OAuth2
- Details
- Category: Spring Boot Microservices
- Mindful Chase By
- Hits: 107
Read more: Event-Driven Architecture in Microservices Using Kafka
- Details
- Category: Spring Boot Microservices
- Mindful Chase By
- Hits: 110
Read more: Spring Cloud Config: Centralized Configuration Management
- Details
- Category: Spring Boot Microservices
- Mindful Chase By
- Hits: 98
Service discovery is a critical component in microservices architecture, enabling seamless communication between services. In a distributed system, services often need to find and interact with each other dynamically. Spring Cloud Netflix Eureka simplifies this by providing a service registry and discovery mechanism. This article explores the core concepts of service discovery, setting up Eureka Server, registering services, and enabling client-side discovery in your Spring Boot application.
Read more: Service Discovery with Spring Cloud Netflix Eureka
- Details
- Category: Spring Boot Microservices
- Mindful Chase By
- Hits: 96
In the world of microservices, ensuring resilience is crucial to maintaining application reliability, especially during failures or latency issues. Resilience patterns like circuit breakers, coupled with tools such as Netflix Hystrix, allow developers to design systems that gracefully degrade rather than fail catastrophically. By implementing these patterns, you can protect your services from cascading failures and improve overall system stability.
Read more: Implementing Resilience with Hystrix and Circuit Breakers
- Details
- Category: Spring Boot Microservices
- Mindful Chase By
- Hits: 92
Effective logging and tracing are essential for debugging and monitoring distributed systems. In a microservices architecture, where services communicate over the network, it becomes critical to track requests as they flow through multiple services. Tools like Spring Sleuth and Zipkin provide robust solutions for adding distributed tracing and logging capabilities to your Spring Boot applications.
Read more: Logging and Tracing in Microservices with Spring Sleuth and Zipkin
- Details
- Category: Spring Boot Microservices
- Mindful Chase By
- Hits: 108
In microservices architecture, an API gateway serves as a crucial intermediary, managing traffic, routing requests, and enforcing security policies. Spring Cloud Gateway, a lightweight and developer-friendly framework, provides a powerful solution for building an API gateway that offers advanced routing and security features out of the box.
Read more: API Gateway with Spring Cloud Gateway: Routing and Security
- Details
- Category: Spring Boot Microservices
- Mindful Chase By
- Hits: 101
Scaling microservices effectively is key to meeting the performance and availability demands of modern applications. By employing horizontal and vertical scaling strategies, you can handle varying workloads while ensuring optimal resource utilization. This article explores these scaling techniques and their application in microservices architectures.
Read more: Scaling Microservices: Horizontal and Vertical Strategies
- Details
- Category: Spring Boot Microservices
- Mindful Chase By
- Hits: 95
Containerization has revolutionized how microservices are developed, deployed, and managed. By using tools like Docker and Kubernetes, you can achieve consistency, scalability, and flexibility in running microservices. This article delves into the fundamentals of containerizing microservices and orchestrating them with Kubernetes.
Read more: Containerizing Microservices with Docker and Kubernetes
- Details
- Category: Spring Boot Microservices
- Mindful Chase By
- Hits: 87
Performance optimization is crucial for microservices, especially as they often handle high workloads and dynamic user demands. In this article, we explore strategies and techniques to enhance the performance of Spring Boot microservices, ensuring low latency, high throughput, and efficient resource utilization.
Read more: Performance Optimization for Spring Boot Microservices
- Details
- Category: Spring Boot Microservices
- Mindful Chase By
- Hits: 94
Managing distributed transactions in a microservices architecture can be challenging. The Saga pattern is a widely used approach to handle these transactions in a consistent and reliable manner. By breaking down a transaction into smaller steps and defining compensating actions for each step, the Saga pattern ensures eventual consistency across services.
Read more: Designing a Saga Pattern Workflow in Distributed Transactions
- Details
- Category: Spring Boot Microservices
- Mindful Chase By
- Hits: 92
Microservices often require a flexible and efficient way to handle complex data operations. The Command Query Responsibility Segregation (CQRS) and Event Sourcing patterns are two architectural approaches that address this need. CQRS separates read and write operations, while Event Sourcing ensures the consistency of data by storing all changes as events.
Read more: Implementing CQRS and Event Sourcing in Microservices
- Details
- Category: Spring Boot Microservices
- Mindful Chase By
- Hits: 95
Reactive programming has become a cornerstone for building scalable and efficient microservices. With Spring WebFlux, developers can create reactive microservices that handle asynchronous, non-blocking I/O, resulting in better performance and resource utilization. This article explores the essentials of building reactive microservices using Spring WebFlux.
Read more: Building Reactive Microservices with Spring WebFlux
- Details
- Category: Spring Boot Microservices
- Mindful Chase By
- Hits: 91
Testing microservices is critical for ensuring reliability, consistency, and seamless integration in distributed systems. A comprehensive testing strategy involves unit, integration, and contract testing to validate the behavior of individual services and their interactions.
Read more: Testing Microservices: Unit, Integration, and Contract Testing
- Details
- Category: Spring Boot Microservices
- Mindful Chase By
- Hits: 95
Securing sensitive data in microservices is paramount to ensure compliance and protect against unauthorized access. By leveraging encryption and tools like HashiCorp Vault in Spring Boot applications, you can safeguard sensitive information such as API keys, passwords, and configuration properties.
Read more: Securing Sensitive Data with Encryption and Vault in Spring Boot
- Details
- Category: Spring Boot Microservices
- Mindful Chase By
- Hits: 101
Monitoring and observability are critical aspects of managing microservices. By using tools like Prometheus and Grafana, you can gain real-time insights into your system's performance and detect issues before they escalate. This article explores how to set up monitoring and observability for microservices with these tools.
Read more: Monitoring and Observability: Using Prometheus and Grafana
- Details
- Category: Spring Boot Microservices
- Mindful Chase By
- Hits: 93
Transitioning from a monolithic architecture to microservices is a strategic move to improve scalability, agility, and maintainability. However, the process involves challenges that require careful planning and execution. This article provides best practices and strategies for successfully migrating a monolithic application to a microservices architecture.
Read more: Migrating Monolith to Microservices: Best Practices and Strategies
- Details
- Category: Spring Boot Microservices
- Mindful Chase By
- Hits: 97
A robust CI/CD pipeline is essential for automating the build, testing, and deployment of microservices. By implementing a CI/CD pipeline for Spring Boot microservices, you can ensure faster delivery, improved quality, and enhanced collaboration. This article outlines the steps to build an effective CI/CD pipeline.
Read more: Building a CI/CD Pipeline for Spring Boot Microservices
- Details
- Category: Spring Boot Microservices
- Mindful Chase By
- Hits: 104
Distributed systems come with inherent challenges, including consistency, latency, and fault tolerance. These challenges arise due to the decentralized nature of microservices and the need for them to work cohesively. This article explores strategies to address these issues and build resilient distributed systems.
Read more: Dealing with Distributed Systems Challenges: Consistency, Latency, and Fault Tolerance
- Details
- Category: Spring Boot Microservices
- Mindful Chase By
- Hits: 81
Spring Cloud provides a powerful suite of tools for building microservices, including advanced capabilities like custom filters and load balancing. These features enable developers to fine-tune request handling and distribute workloads efficiently, ensuring reliability and scalability in microservices architectures.
Read more: Advanced Spring Cloud: Custom Filters and Load Balancing